Full Stack
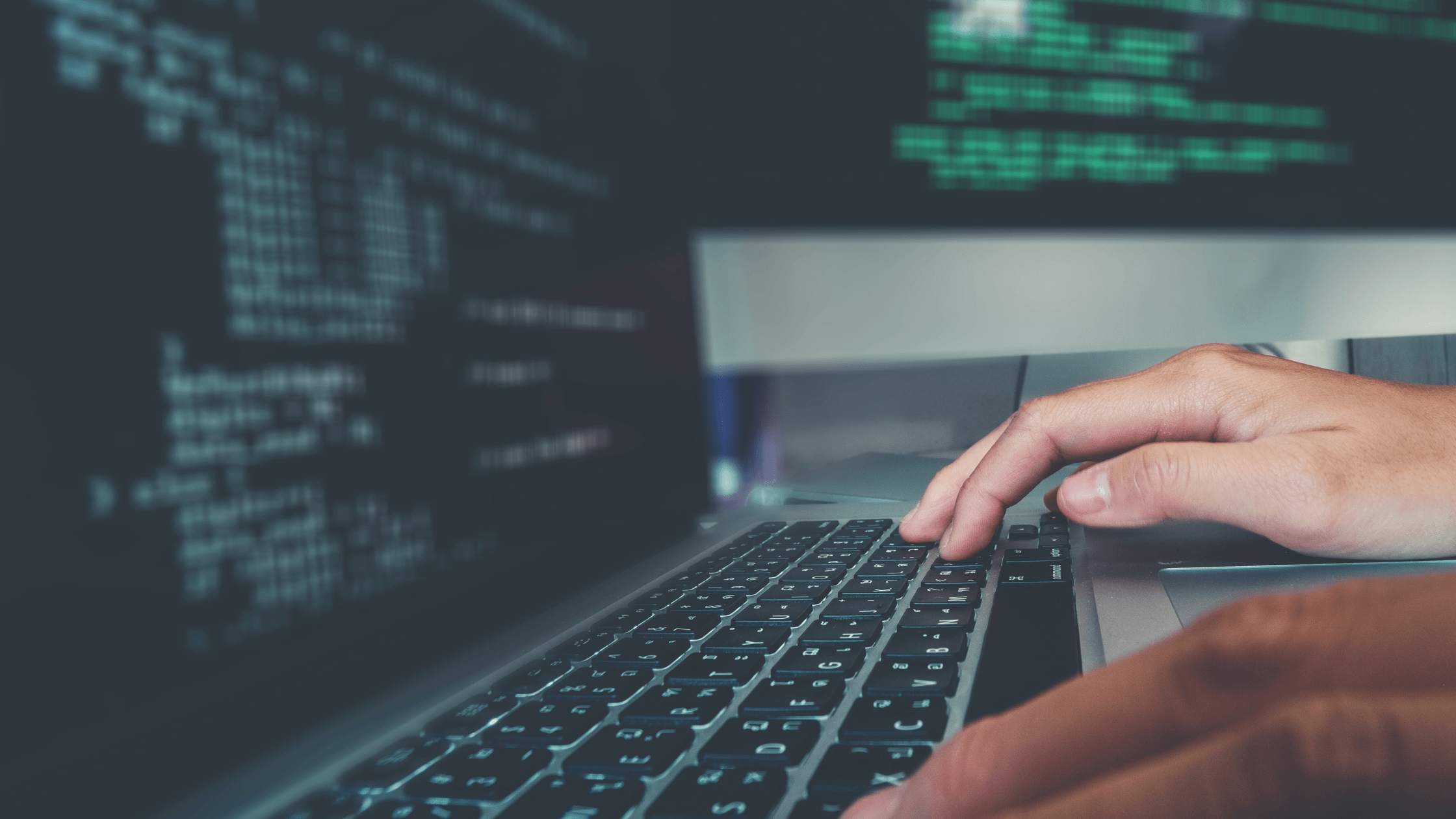
Full Stack
Explore our comprehensive Full Stack Application Development course, centered around Python, Flask, and React technologies. Gain in-depth insights and hands-on experience for mastering the art of building robust applications.
Course Details
1. Module
1. Introduction to Python
2. Python Data Structures
– Lists
– Dictionaries
– Strings
– Tuples
3. Modules in Python
– Creating applications using built-in modules
– Creating applications using third-party modules
4. Functions
– Developing functions in Python
– Creating your own module
– Overloading of functions
5. Object-Oriented Coding in Python
– Overriding the magic methods
– Understanding inheritance
2. Module – Backend Development: SQL
- Introduction to SQL
- Exploring Popular Technologies: SQLite3, MongoDB
- Database Creation and Table Setup
- Understanding the Concept of Primary Key and Foreign Key
- Data Manipulation
- Inserting Data into Tables using the INSERT Command
- Fetching Data from Tables using the SELECT Command
- Filtering Data using the WHERE Clause
- Understanding Joins in SQL
Module 3 – Backend Development: Flask
1. Understanding Flask and Its Importance
2. Flask vs. Other Frameworks (e.g., Django)
3. Building Your First Web Application with Flask
4. Implementing API Functionality
– Handling HTTP Methods: GET, POST, PUT, DELETE
5. Connecting with the Datastore
6. Implementing Object-Relational Mapping (ORM) with SQLAlchemy
7. Authentication and Authorization
8. Utilizing JWT Tokens for Security
Module 1 – Frontend Development: HTML and CSS
1. HTML and CSS Fundamentals
Module 2 – Frontend Development: JavaScript
1. Introduction to JavaScript
2. JavaScript vs TypeScript
3. Understanding NodeJS
4. Variable Declaration: let vs const
5. Functionality in JavaScript
– Writing Functions
– Exploring Arrow Functions
– Working with Arrays: map, filter, sort, for Each
6. Promises in JavaScript
7. Asynchronous Communication with async and await
Module 3 – Frontend Development: React
1. Introduction to React and Its Popularity
2. Building Your First React Application
3. Understanding React Hooks: useState and useEffect
4. React Routings
5. Data Retrieval from the Server
– Utilizing Fetch and Axios
6. Event Handling in React